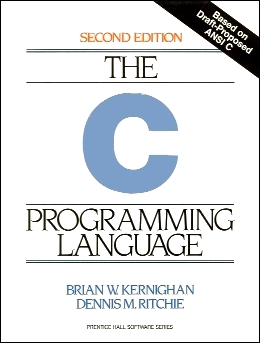
Below you can find the documentation of C library functions.
/********************************************************************************
* Try to connect to the device
* Returns: lwHandle for success, 0 for fail
********************************************************************************/
littleWire* littleWire_connect();
/*******************************************************************************/
/********************************************************************************
* Returns the firmware version
* Format: 0xXY => X: Primary version Y: Minor version
********************************************************************************/
unsigned char readFirmwareVersion(littleWire* lwHandle);
/*******************************************************************************/
/********************************************************************************
* Set a GPIO pin High/Low
* pin: Pin number
* state: 1 for High, 0 for Low
********************************************************************************/
void digitalWrite(littleWire* lwHandle, unsigned char pin, unsigned char state);
/*******************************************************************************/
/********************************************************************************
* Set a GPIO pin input/output
* pin: Pin number
* mode: 1 for input, 0 for output
********************************************************************************/
void pinMode(littleWire* lwHandle, unsigned char pin, unsigned char mode);
/*******************************************************************************/
/********************************************************************************
* Read a state of a GPIO pin
* pin: Pin number
* Returns: 1 for HIGH, 0 for LOW
********************************************************************************/
unsigned char digitalRead(littleWire* lwHandle, unsigned char pin);
/*******************************************************************************/
/********************************************************************************
* Read analog voltage from a spesific channel
* channel: 0 for pin3, 1 for pin2, 2 for internal Temperature sensor
* Returns: Analog voltage in 10bit resoultion
********************************************************************************/
unsigned int analogRead(littleWire* lwHandle, unsigned char channel);
/*******************************************************************************/
/********************************************************************************
* Initialize the Pwm module on the device
********************************************************************************/
void pwm_init(littleWire* lwHandle);
/*******************************************************************************/
/********************************************************************************
* Stop the PWM module on the device
********************************************************************************/
void pwm_stop(littleWire* lwHandle);
/*******************************************************************************/
/********************************************************************************
* Update the compare values of Pwm outputs
* channelA: Compare value of Channel A
* channelB: Compare value of Channel B
/*******************************************************************************/
void pwm_updateCompare(littleWire* lwHandle, unsigned char channelA, unsigned char channelB);
/*******************************************************************************/
/********************************************************************************
* Change the Pwm prescaler. Default: 1024
* value: 1024/256/64/8/1
********************************************************************************/
void pwm_updatePrescaler(littleWire* lwHandle, unsigned int value);
/*******************************************************************************/
/********************************************************************************
* Initialize SPI module
********************************************************************************/
void spi_init(littleWire* lwHandle);
/*******************************************************************************/
/********************************************************************************
* Send one byte SPI message. Chip select is manual.
* message: Message to send
* Returns: Received SPI message
********************************************************************************/
unsigned char spi_sendMessage(littleWire* lwHandle, unsigned char message);
/*******************************************************************************/
/********************************************************************************
* Send multiple SPI messages. Chip select is manual/automatic.
* sendBuffer: Message array to send
* inputBuffer: Returned answer message
* length: Message length - maximum 4
* mode: 1 for auto chip select , 0 for manual
********************************************************************************/
void spi_sendMessage_multiple(littleWire* lwHandle, unsigned char * sendBuffer, unsigned char * inputBuffer, unsigned char length ,unsigned char mode);
/*******************************************************************************/
/********************************************************************************
* Update SPI signal delay amount. Tune if neccessary to fit your requirements.
* duration: Delay amount.
********************************************************************************/
void spi_updateDelay(littleWire* lwHandle, unsigned int duration);
/*******************************************************************************/
/********************************************************************************
* Initialize i2c module on Little-Wire
********************************************************************************/
void i2c_init(littleWire* lwHandle);
/*******************************************************************************/
/********************************************************************************
* Start the i2c tranmission
* address: Slave device address
********************************************************************************/
void i2c_beginTransmission(littleWire* lwHandle, unsigned char address);
/*******************************************************************************/
/********************************************************************************
* Add new byte to the i2c send buffer
* message: A byte to send.
********************************************************************************/
void i2c_send(littleWire* lwHandle,unsigned char message);
/*******************************************************************************/
/********************************************************************************
* Send the whole message buffer to the slave at once and end the tranmssion.
********************************************************************************/
void i2c_endTransmission(littleWire* lwHandle);
/*******************************************************************************/
/********************************************************************************
* Request a reply / message from a slave device.
* address: Slave address
* numBytes: Number of bytes the slave will send.
* responseBuffer: Array pointer which will hold the response from the slave
********************************************************************************/
void i2c_requestFrom(littleWire* lwHandle,unsigned char address,unsigned char numBytes,unsigned char * responseBuffer);
/*******************************************************************************/